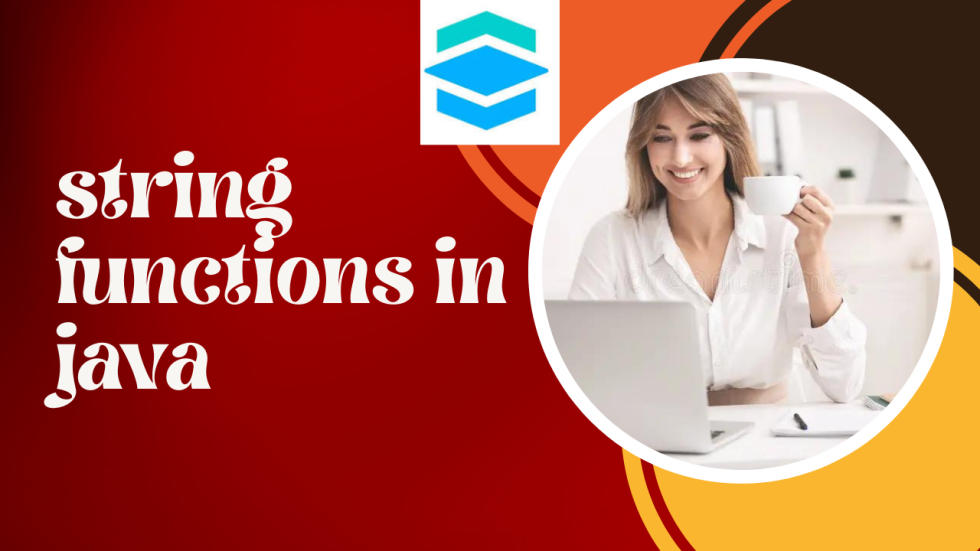
Unraveling the Magic of Java: A Journey into Object Class and String Functions
Java, the stalwart of programming languages, boasts an extensive library of features and functionalities. Among these, the Object Class in Java and String Functions in Java stand out as crucial components that empower developers to create robust and dynamic applications. In this exploration, we will delve into the intricacies of these two elements, unlocking their potential and understanding their significance in the Java programming landscape.
The Foundation: Understanding Object Class in Java
The Object Class serves as the foundation for all Java classes, forming the root of the class hierarchy. Every class in Java is a direct or indirect derivative of the Object Class. Let’s unravel the magic behind this fundamental concept.
1. Essence of Object Class
At its core, the Object Class encapsulates the basic functionalities that are inherent to all Java objects. From methods like toString()
to equals()
and hashCode()
, it provides a blueprint that subclasses can build upon. This intrinsic connection between every Java class and the Object Class establishes a unified structure within the language.
2. Inheritance: The Backbone of Object Class
In the realm of Java programming, inheritance plays a pivotal role. The Object Class, being the pinnacle of this hierarchy, imparts its methods and attributes to all its descendants. This inheritance mechanism ensures a standardized approach to essential operations, promoting consistency and coherence across diverse codebases.
3. Polymorphism and Object Class
Polymorphism, a cornerstone of object-oriented programming, finds a natural ally in the Object Class. By embracing polymorphism, developers can write code that interacts with objects of various classes, all rooted in the common ancestry of the Object Class. This flexibility enhances code reuse and fosters a modular programming paradigm.
4. Object Class Methods in Action
Let’s illustrate the practicality of Object Class methods with a simple example:
javaCopy code
public class CustomObject { private String identifier; // Constructor and other class-specific methods @Override public String toString() { return "CustomObject - Identifier: " + identifier; } } // Usage CustomObject customObj = new CustomObject(); System.out.println(customObj.toString());
In this snippet, the toString()
method inherited from the Object Class is overridden to provide a custom representation of the CustomObject
. This showcases how the Object Class methods can be tailored to suit the needs of individual classes.
Navigating the String Functions in Java
While the Object Class lays the groundwork for Java classes, String Functions empower developers to manipulate text effectively. Let’s embark on a journey through the diverse and powerful world of string operations in Java.
5. The Power of Strings
Strings in Java are more than just sequences of characters; they are dynamic entities with an array of built-in functions. From concatenation to substring extraction, these String Functions offer a versatile toolkit for handling textual data.
6. Concatenation: Joining Strings with Ease
One of the most basic yet essential String Functions is concatenation. The concat()
method allows developers to seamlessly join two strings, facilitating the creation of composite strings.
javaCopy code
String firstName = "John"; String lastName = "Doe"; String fullName = firstName.concat(" ").concat(lastName);
In this example, the concat()
method brings together the firstName
and lastName
strings, forming the complete fullName
. Such operations are fundamental in various scenarios, from constructing user interfaces to generating dynamic content.
For more interesting content, Visit newsonclicks.com
7. Length Matters: String Length Function
Determining the length of a string is a common requirement in programming. Java provides the length()
method, enabling developers to retrieve the number of characters in a given string effortlessly.
javaCopy code
String message = "Hello, Java!"; int length = message.length(); System.out.println("Length of the string: " + length);
Here, the length()
method accurately computes and outputs the length of the message
string, which is crucial for tasks like input validation and memory allocation.
8. Substring Extravaganza
String manipulation often involves extracting substrings based on specific criteria. Java offers the substring()
method, allowing developers to carve out portions of a string with precision.
javaCopy code
String sentence = "Java programming is fascinating!"; String substring = sentence.substring(5, 16); System.out.println("Extracted Substring: " + substring);
In this example, the substring()
method isolates the substring from the 5th to the 16th index of the sentence
, showcasing the finesse of string manipulation in Java.
# Weaving Object Class and String Functions Together: A Harmonious Symphony
Now that we have explored the individual realms of Object Class and String Functions, let’s unravel the magic that unfolds when these two components harmonize in Java development.
9. Object Class and String Representation
When crafting classes, it’s common to leverage the Object Class’s toString()
method to provide a meaningful string representation of an object. This is where the synergy with String Functions comes into play.
javaCopy code
public class Product { private String name; private double price; // Constructor and other class-specific methods @Override public String toString() { return "Product: " + name + " | Price: $" + price; } } // Usage Product laptop = new Product("Laptop", 999.99); System.out.println(laptop.toString());
In this scenario, the toString()
method of the Product
class utilizes String concatenation to construct a detailed string representation, incorporating the name and price of the product.
For more interesting content, Visit newsonclicks.com
10. String Functions in Object Class Methods
Consider a scenario where a class needs to manipulate strings as part of its functionality. By incorporating String Functions within the methods of that class, developers can enhance the versatility of their code.
javaCopy code
public class StringManipulator { public static String reverse(String input) { return new StringBuilder(input).reverse().toString(); } } // Usage String original = "Java Programming"; String reversed = StringManipulator.reverse(original); System.out.println("Reversed String: " + reversed);
Here, the StringManipulator
class employs the reverse()
method to reverse a given string, showcasing the seamless integration of String Functions within Java classes.
# Conclusion: Unleashing the Potential of Java Programming
In the vast landscape of Java programming, understanding the nuances of the Object Class and String Functions is paramount. These elements, though distinct, share a symbiotic relationship, contributing to the elegance and efficiency of Java code.
As we conclude this exploration, it’s evident that the Object Class forms the bedrock of Java’s class hierarchy, promoting code consistency through inheritance and polymorphism. Simultaneously, String Functions provide a dynamic toolkit for manipulating textual data, adding a layer of versatility to Java’s capabilities.
Whether you’re crafting intricate class hierarchies or sculpting strings with finesse, the synergy between the Object Class and String Functions epitomizes the magic that unfolds within the realm of Java programming. Embrace these elements, master their intricacies, and unlock the full potential of Java as a programming language.
By ScholarHat